game maker studio draw 3d object
When people ask questions about 3D limitations in GameMaker, it'south usually in the context of "tin can I make a AAA MMO with HDR and 4K textures?". But occasionally, people will inquire about making two.5D projects similar to Wolfenstein, and e'er so oftentimes someone comes along that has actually decided to make a fully 3D game.
One particular game genre that has always existed on the sidelines but with a loyal following tin can specially do good from 3D rendering. No need for circuitous collision, geometry, animation or fancy camera movement – these games can utilize 3D for moody fog and basic lighting, or even just to help generate environments without having to hand-depict 3D projections.
I'm of form talking about CRPGs, and one of the earlier forms of CRPGs were showtime person dungeon crawlers, using either 3D or traditional 2D graphics.
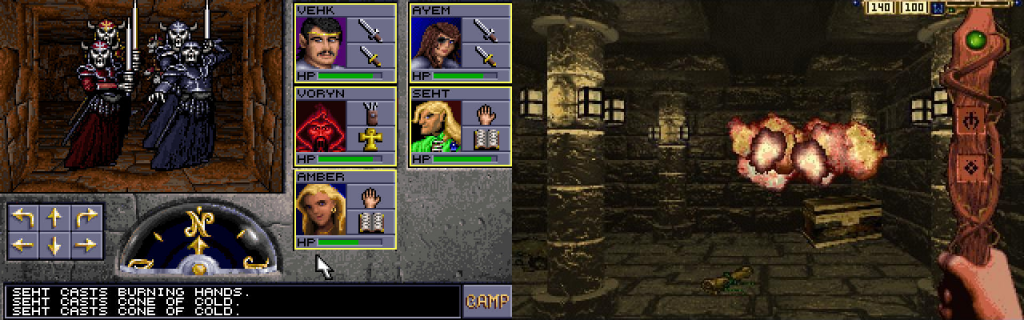
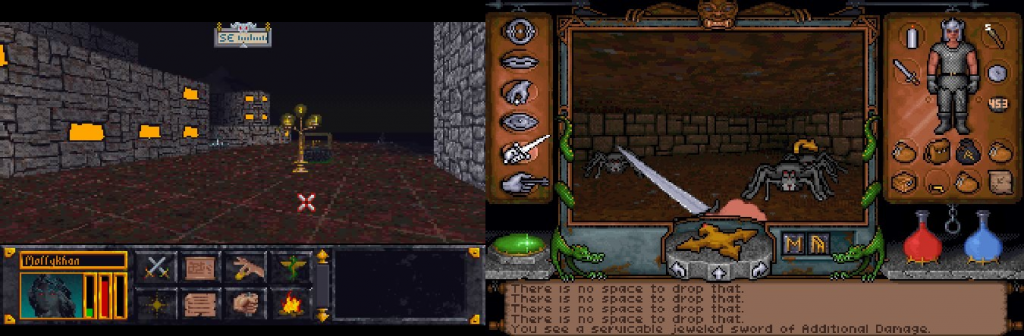
As a game designer, I oft find myself walking a tightrope of minimizing the attempt needed to establish an art pipeline for a game and getting a desired aesthetic. 3D takes away the need for drawing 2D art in perspective, but adds many other complications. However, the results are frequently satisfying.
Setting up
For the purpose of this tutorial, the instructions will exist as simple as possible. I'll be using the GMS2 room editor, but as your telescopic and agreement increases, you may want (or have) to write your own tool for building 3D worlds.
Y'all tin can discover the companion GMS2 project for this tutorial here (.zippo). I volition be referring to this project throughout the tutorial.
I'm going to presume you lot're familiar with the room editor, and so the parts I will focus on are the scripts and functions needed to catechumen a collection of 2D data to a format usable in 3D and the process of rendering it as a 3D scene. In this case, we'll exist working with a ds_grid converted from tiles that tin can be placed in the room editor.
Converting placed tiles to a grid
I am including this because not all users are familiar with tiles – if you'd like to write your own method of gathering data on where to brand world geometry, experience costless to skip this part.
/// @func layer_tilemap_to_grid /// @desc {void} takes a given tile layer and populates a given ds_grid with a given value /// @arg {cord} layerName /// @arg {grid} grid /// @arg {any} value var _layerName = statement[0]; var _grid = statement[one]; var _value = statement[2]; var _layer = layer_get_id(_layerName); var _tileMap = layer_tilemap_get_id(_layer); var _width = ds_grid_width(_grid) var _height = ds_grid_height(_grid) var _tile = -1; for (var _y = 0; _y < _height ; _y++){ for (var _x = 0; _x < _width; _x++){ _tile = tilemap_get(_tileMap, _x, _y); if (_tile == 1){ _grid[# _x, _y] = _value; } } }
This goes through a grid loop and checks to meet if a tile of index 1 exists at a given position on the tilemap, and so sets that position in a companion grid. Grids are easier to access than tilemaps and in that location are many places in the case projection where grid checks are performed.
Now that we take our data in grid format, let's motility on to the interesting stuff.
Overview: understanding the 3D pipeline
In society to build our world and return it on screen, nosotros demand to practise a few things:
one) Define a vertex format
The vertex format needs to exist defined showtime, setting the rules of how the buffer is constructed and rendered.
2) Build a vertex buffer
A vertex buffer is the collection of vertex data – it is where we build our world.
3) Set a texture
Setting the texture specifies the epitome mapped to geometry, only it's not the same thing as a sprite asset. Textures are defined in lawmaking using sprite_get_texture(sprite_index, image_index). The texture can be made up of many images of varying sizes, but we'll talk a scrap about how textures are mapped onto geometry later.
4) Submit the vertex buffer
Finally, a vertex buffer can be rendered using vertex_submit(vertex buffer, primitive type, texture), either in a surface or a draw event. When submitting the vertex buffer, a texture should be specified unless you opt to but use a vertex colour instead. In that case, you can safely pass in -ane every bit the texture.
Now, permit's get into the details of each step.
Defining a vertex format
A vertex format determines how the vertex buffer is built and interpreted by the renderer. It needs position and color information at minimum, but texcoords are required if y'all want to display textures over geometry. Vertex formats can be defined at any time earlier you start to build the vertex buffer, and so I put mine in the create event of a controller object.
vertex_format_begin(); vertex_format_add_position_3d(); vertex_format_add_texcoord(); vertex_format_add_colour(); world_format = vertex_format_end();
Building a vertex buffer (from a grid)
This is the fun part! We need to iterate over a grid one time to build each cell of our grid into world geometry.
for (var _gridY = 0; _gridY < world_height; _gridY++){ for (var _gridX = 0; _gridX < world_width; _gridX++){ world_build_cell(_gridX, _gridY); } }
world_build_cell(_gridX, _gridY);
for (var _i = 0; _i < __WALL.SIZE; _i++){ var _empty = global.world_grid[# _gridX, _gridY] == __CELL.EMPTY; if (_empty){ world_build_wall(_gridX, _gridY, _i); } }
This is where we build the actual geometry. For each cell, we need to build up to six walls, then we loop through the size of our wall enum(0-5) and send that value in.
From now on, when we're talking about geometry, we'll apply the floor as our instance, because it is the easiest to sympathise. If you compare it with the other wall building code in the sample project, y'all may notice some patterns. If you exercise not conform to these patterns when building geometry, you will become alternative and texture errors on return.
First we'll look at the geometry:
// Tri ane vertex_position_3d(world_vbuff, _x, _y, _z); // Top Left vertex_position_3d(world_vbuff, _x2, _y, _z); // Top Right vertex_position_3d(world_vbuff, _x2, _y2, _z); // Bot Correct // Tri 2 vertex_position_3d(world_vbuff, _x, _y, _z); // Top Left Again vertex_position_3d(world_vbuff, _x2, _y2, _z); // Bot Right Once again vertex_position_3d(world_vbuff, _x, _y2, _z); // Bot Left
The guild triangles are congenital in does not thing, but the lodge their vertices are built in does. The cullmode we use for this project is anti-clockwise, so all triangles congenital clockwise will return from the "front", and all triangles built anti-clockwise volition return from the "back".
As an alternative to manually defining all six walls, information technology is too possible to build 2 triangles six times and rotate each pair, simply that is exterior the scope of this tutorial.
When building these triangles, we accept to populate the vertex data in accordance with the format, so you can't only list out all of the geometry and then all of the colour data – it makes for some messy lawmaking.
vertex_position_3d(world_vbuff, _x, _y, _z); vertex_texcoord(world_vbuff, texcoord_floor_u, texcoord_floor_v); vertex_colour(world_vbuff, _colour, i);
Yous need all three of these functions to properly build in the provided format. vertex_colour is simply telling the renderer to return that vertex at 100% blastoff and 100% brightness with no hue changes. Simply what about vertex_texcoord?
Setting up texture coordinates
Texcoords are another type of coordinate system, this time normalised (0 through 1). A texcoord can be understood as the coordinate pair on a texture where a particular prototype starts or stops. You tin can use these to perfectly align textures or stretch and squash them.
Using the instance texture for this tutorial, you tin can see these coordinates are y-down. This might take some getting used to if you've ever spent whatever time reading middle school math textbooks. Coincidentally, GameMaker is likewise y-downwards.
Texcoords are labeled with u and v. u,v is analogous to x,y.
If we place four images on our sprite for use as our texture, and so nosotros need to remember where each image begins. I do the following for quick reference but it isn't necessary.
texcoord_size = 0.5; // normalised infinite all our sprites take upward on our texture texcoord_wall_u = 0.five; texcoord_wall_v = 0; texcoord_floor_u = 0.5; texcoord_floor_v = 0.5; texcoord_ceiling_u = 0; texcoord_ceiling_v = 0;
These coordinates are inserted into the vertex buffer later on our position data, just for the sake of clarity, I have removed all other vertex functions from this case.
// Tri 1 vertex_texcoord(world_vbuff, texcoord_floor_u, texcoord_floor_v); vertex_texcoord(world_vbuff, texcoord_floor_u + texcoord_size, texcoord_floor_v); vertex_texcoord(world_vbuff, texcoord_floor_u + texcoord_size, texcoord_floor_v + texcoord_size); // Tri two vertex_texcoord(world_vbuff, texcoord_floor_u, texcoord_floor_v); vertex_texcoord(world_vbuff, texcoord_floor_u + texcoord_size, texcoord_floor_v + texcoord_size); vertex_texcoord(world_vbuff, texcoord_floor_u, texcoord_floor_v + texcoord_size);
Setting upward a view and projection matrix
Before nosotros tin render anything on screen, we need to tell the renderer how to approach the scene. The default return style in GameMaker is a 2d top-down orthographic view: there is no perspective involved in this type of view, so we'll need to fix that up.
Many of the topics I've covered in this tutorial could accept an unabridged book written about them, and this step is no exception. Because merely a very unproblematic photographic camera is necessary, I will exist providing some code every bit-is and very briefly explaining what values are for what. If yous would like to learn more virtually projection matrices view the resources at the bottom of this tutorial.
In GameMaker, the render matrix variables (view, projection and world) directly affect the application surface – no demand for messy camera or view setups! If I say "camera" or "view" from at present on, I am speaking in general terms, not well-nigh GameMaker specific tools.
var _camX = _playerX; var _camY = _playerY; var _camZ = _playerZ; var _camDX = dcos(_playerDir); var _camDY = -dsin(_playerDir); matrix_set(matrix_view, matrix_build_lookat(_camX, _camY, _camZ, _camX + _camDX, _camY + _camDY, _camZ, 0, 0, i));
You can think of the view matrix (matrix_view) as the position of a camera. The arguments are divided into three sets: From [X,Y,Z], To [Ten,Y,Z], and Upwardly [X,Y,Z]. Because we are rendering a Z-up world in this example, the third set of arguments, Up, is [0,0,i]. Information technology is also mutual to have a Y-upward world, using [0,i,0] instead.
The From and To arguments can exist identical, except if yous want to rotate your photographic camera; in that example, you'll demand to add together a normalised value (-1 through ane) to the To position.
matrix_set(matrix_projection, matrix_build_projection_perspective_fov(ninety, room_width / room_height, 1, 2000));
If the view matrix is the camera property representing its position, then the projection matrix is the camera property representing its lens. The kickoff argument is FOV, the next our aspect ratio (4:iii), and finally the nigh and far clip. The nearly prune is where the rendering starts, and the far prune is where the rendering stops, in relation to the camera position. Virtually clip should be a positive non-zippo value or you will take depth sorting bug. Far prune can be whatever you similar.
Rendering the world
So far this has been a lot of setup with no payoff, so let's prepare that! After you've converted your data to be readable, built geometry from information technology, and fix up your matrices, information technology is time for the moment of truth… In a surface or describe event of your choosing, submit your vertex buffer!
vertex_submit(world_vbuff, pr_trianglelist, world_texture);
Take note of the brake of one texture per buffer – the reason why all images have to exist on the same texture should make more sense now. You lot can return as many buffers as your GPU tin handle, merely y'all should still try to avoid quadratic loops and rendering each cell equally its own buffer.
If you lot look over the source code, y'all'll see a lot of fiddling details get added in here and there, render fog being 1 of them. Bank check it out and experiment with calculation more circuitous features!
Resources
This stuff is miles deep, merely for the most basic applications, you won't need to read any of it.
Bronze Box repo, may be updated in the hereafter
https://github.com/cicadian/Bronze-Box/
Basically everything you need to exist a (3D) professional video game developer cosplayer
https://learnopengl.com/
https://www.scratchapixel.com/lessons/mathematics-physics-for-computer-graphics/geometry
3D Projection
https://en.wikipedia.org/wiki/3D_projection
https://world wide web.scratchapixel.com/lessons/3D-basic-rendering/rendering-3D-scene-overview/perspective-projection
Rotation of geometry
https://learnopengl.com/Getting-started/Transformations
https://en.wikipedia.org/wiki/Rotation_matrix
Therapy for later when you want to larn lighting and model importing
https://www.google.com/search?&q=pictures+of+cats
twitter.com/jujuadams
Source: https://deerbell.games/blog/basic-2d-3d-projection-using-gamemaker/
0 Response to "game maker studio draw 3d object"
Yorum Gönder